Hi,
Its very interesting to create iPhone Like AlertView (Dialog Box) in Android.
Follow the steps to create iPhone Like AlertView (Dialog Box) in Android
Step 1 : Create android Project in Eclipse as shown below
Step 2 : Create New Class for Custom Dialog as given below
After creating CustomizeDialog.java write following code into it.
package com.sks.dialog.custom;
import android.app.Dialog;
import android.content.Context;
import android.text.method.ScrollingMovementMethod;
import android.view.View;
import android.view.View.OnClickListener;
import android.view.Window;
import android.widget.Button;
import android.widget.TextView;
/** Class Must extends with Dialog */
/** Implement onClickListener to dismiss dialog when OK Button is pressed */
public class CustomizeDialog extends Dialog implements OnClickListener {
Button okButton;
Context mContext;
TextView mTitle = null;
TextView mMessage = null;
View v = null;
public CustomizeDialog(Context context) {
super(context);
mContext = context;
/** 'Window.FEATURE_NO_TITLE' - Used to hide the mTitle */
requestWindowFeature(Window.FEATURE_NO_TITLE);
/** Design the dialog in main.xml file */
setContentView(R.layout.main);
v = getWindow().getDecorView();
v.setBackgroundResource(android.R.color.transparent);
mTitle = (TextView) findViewById(R.id.dialogTitle);
mMessage = (TextView) findViewById(R.id.dialogMessage);
okButton = (Button) findViewById(R.id.OkButton);
okButton.setOnClickListener(this);
}
@Override
public void onClick(View v) {
/** When OK Button is clicked, dismiss the dialog */
if (v == okButton)
dismiss();
}
@Override
public void setTitle(CharSequence title) {
super.setTitle(title);
mTitle.setText(title);
}
@Override
public void setTitle(int titleId) {
super.setTitle(titleId);
mTitle.setText(mContext.getResources().getString(titleId));
}
/**
* Set the message text for this dialog's window.
*
* @param message
* - The new message to display in the title.
*/
public void setMessage(CharSequence message) {
mMessage.setText(message);
mMessage.setMovementMethod(ScrollingMovementMethod.getInstance());
}
/**
* Set the message text for this dialog's window. The text is retrieved from the resources with the supplied
* identifier.
*
* @param messageId
* - the message's text resource identifier <br>
* @see <b>Note : if resourceID wrong application may get crash.</b><br>
* Exception has not handle.
*/
public void setMessage(int messageId) {
mMessage.setText(mContext.getResources().getString(messageId));
mMessage.setMovementMethod(ScrollingMovementMethod.getInstance());
}
}
Very Important part to hide dialog default background.
below code is used to set transparant background of dialog.
v = getWindow().getDecorView();
v.setBackgroundResource(android.R.color.transparent);
Step 3 : Now We have to create custom Layout for Dialog.
edit main.xml (you can select layout file name as per your specifications)
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/LinearLayout"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:layout_margin="0dip"
android:background="@drawable/alert_bg"
android:orientation="vertical"
android:paddingBottom="15dip"
android:paddingLeft="0dip"
android:paddingRight="0dip"
android:paddingTop="0dip" >
<TextView
android:id="@+id/dialogTitle"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_marginTop="20dip"
android:background="#00000000"
android:gravity="center"
android:text=""
android:textColor="#fff"
android:textSize="22sp"
android:textStyle="bold" >
</TextView>
<TextView
android:id="@+id/dialogMessage"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_below="@+id/dialogTitle"
android:focusable="true"
android:focusableInTouchMode="true"
android:gravity="center"
android:maxLines="10"
android:padding="10dip"
android:scrollbars="vertical"
android:text=""
android:textColor="#fff"
android:textSize="18sp" >
</TextView>
<Button
android:id="@+id/OkButton"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_below="@+id/dialogMessage"
android:layout_centerHorizontal="true"
android:layout_marginLeft="10dip"
android:layout_marginRight="10dip"
android:background="@drawable/button"
android:text="OK"
android:textColor="#FFFFFF" >
</Button>
</RelativeLayout>
Step 4 : Add 9 patch image in res->drawable folder given below. You can modify image.
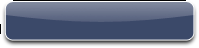 |
alert_bg.9.png |
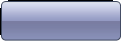 |
button_bg_normal.9.png |
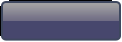 |
button_bg_pressed.9 |
 |
titlebg.png
Note : this image transparency is 90% that's why invisible |
Step 5 : For adding Focus Effect on Dialog Button create button.xml in drawable folder as given below
<?xml version="1.0" encoding="utf-8"?>
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<item android:state_pressed="true" android:drawable="@drawable/button_bg_pressed" /> <!-- pressed -->
<item android:state_focused="true" android:drawable="@drawable/button_bg_pressed" /> <!-- focused -->
<item android:drawable="@drawable/button_bg_normal" /> <!-- default -->
</selector>
Step 6 : Now Come to our First Activity i.e. CustomDialogExample.java and Modify file as given Below
package com.sks.dialog.custom;
import android.app.Activity;
import android.content.Context;
import android.os.Bundle;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
public class CustomDialogExample extends Activity {
Context context;
CustomizeDialog customizeDialog = null;
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
/** Display Custom Dialog */
context = this;
setContentView(R.layout.home);
Button btn1 = (Button) findViewById(R.id.btnDialog1);
btn1.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
customizeDialog = new CustomizeDialog(context);
customizeDialog.setTitle("My Title");
customizeDialog.setMessage("My Custom Dialog Message from \nSmartPhoneBySachin.Blogspot.com ");
customizeDialog.show();
}
});
}
}
Now you are ready to Run Project and you will get result as image below
Download Source Code and Installer Apk
Comments are always welcome.
Enjoy Customization in Android